Let’s say you have a certain product that you need to capture additional information for at checkout. Using WooCommerce we can add fields to the checkout based on nearly any conditional matches.
For this tutorial we will be using the premise of a book seller. Let’s say that the store owner wants to add an option at checkout for the user to choose whether they would like the book inscribed with a message from the author.
Adding Custom Fields to Checkout
WooCommerce is filled with many useful hooks to tie into with our code. The checkout action we will be using for this tutorial is woocommerce_after_order_notes which will allow us to add our custom fields directly below the “Order Notes” field in the checkout form. If you would like your fields to display elsewhere there are a number of additional hooks you can use such as woocommerce_after_shipping_calculatorand woocommerce_after_shop_loop OR woocommerce_after_shipping_calculatorand woocommerce_before_shop_loop.
Within the following function we will check what items the user has in their cart by looping through their products and flagging a variable as true or false. If the variable is false the fields will not output, if it is true and the specific product is in the cart will will output our fields. Here’s the code:
/*----------------------------------------------------------------------------------- START*/
/* Extra fields | InCreativeWeb
REF - https://wordimpress.com/create-conditional-checkout-fields-woocommerce/
/*-----------------------------------------------------------------------------------*/
/** [1] Add the field to the checkout **/
add_action( 'woocommerce_before_order_notes', 'icw_custom_checkout_field' );
function icw_custom_checkout_field( $checkout ) {
//Check if Book in Cart (UPDATE WITH YOUR PRODUCT ID)
// http://chakaboomfitness.com/wp-admin/post.php?post=1235&action=edit
$ticket_pickup_in_cart = icw_is_conditional_product_in_cart( 1235 ); // enter product id @icw
//Book is in cart so show additional fields
if ( $ticket_pickup_in_cart === true ) {
echo '< div class="icw-block" >< h3 >' . __( 'Ticket(s) Pickup *' ) . '';
woocommerce_form_field( 'ticket-pickup', array(
'type' => 'radio',
'class' => array( 'icw-ticket-pickup form-row-wide icw-radio-inline' ),
//'label' => __( '' ),
'required' => true,
'default' => 'I will pick up at Franconia',
'options' => array('I will pick up at Franconia' => 'I will pick up at Franconia', 'Please mail the tickets to me' => 'Please mail the tickets to me')
), $checkout->get_value( 'ticket-pickup' ) );
woocommerce_form_field( 'if-checked-address-tickets', array(
'type' => 'textarea',
'class' => array( 'icw-textarea-hide form-row-wide' ),
'label' => __( 'Please enter address to mail your tickets' ),
), $checkout->get_value( 'if-checked-address-tickets' ) );
woocommerce_form_field( 'if-checked', array(
'type' => 'checkbox',
'class' => array( 'icw-checkbox form-row-wide if-checked' ),
'value' => 'Yes',
'label' => __( 'Check if anyone attending is under 21 (Anyone under 21 must be accompanied by legal guardian).' ),
), $checkout->get_value( 'if-checked' ) );
woocommerce_form_field( 'if-checked-under-21', array(
'type' => 'textarea',
'class' => array( 'icw-textarea-hide form-row-wide' ),
'label' => __( 'Please enter how many under 21 will be attending' ),
), $checkout->get_value( 'if-checked-under-21' ) );
echo '';
?>< style type="text/css" >
.icw-block { background: #fff; padding: 15px; margin-bottom:20px !important; border-radius: 10px; border: 3px solid #25b60c;}
.icw-textarea-hide { display:none; }
.icw-radio-inline label.radio {display:inline-block !important; padding:0px 20px 0px 5px}
< script >
jQuery(document).ready(fun ction($){
$('.icw-checkbox input[type="checkbox"]').on('click', function () {
$('#if-checked-under-21_field').slideToggle();
});
$('.icw-ticket-pickup input[type=\'radio\']').change(function(){
if($('.icw-ticket-pickup .input-radio:last').is(':checked')){
$('#if-checked-address-tickets_field').slideDown();
}else{
$('#if-checked-address-tickets_field').slideUp();
}
});
});
< ?php
}
}
/** [2] Check if Conditional Product is In cart @param $product_id */
function icw_is_conditional_product_in_cart( $product_id ) {
//Check to see if user has product in cart
global $woocommerce;
//flag no book in cart
$ticket_pickup_in_cart = false;
foreach ( $woocommerce->cart->get_cart() as $cart_item_key => $values ) {
$_product = $values['data'];
if ( $_product->id === $product_id ) {
//book is in cart!
$ticket_pickup_in_cart = true;
}
}
return $ticket_pickup_in_cart;
}
Here’s what my checkout fields look like with some additional styling.
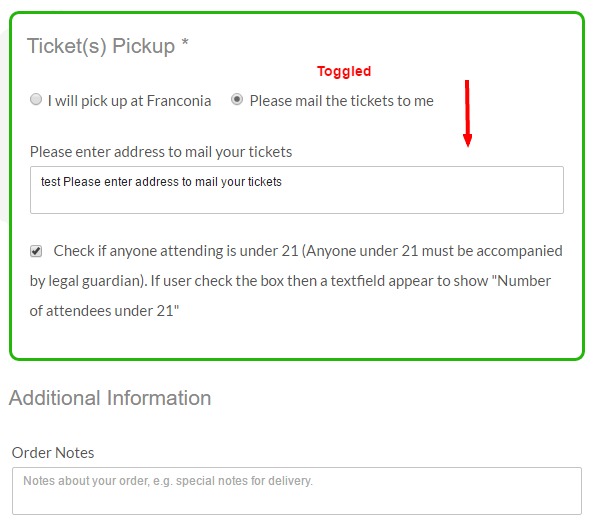
Tip: Check out the woocommerce_form_field function within WooCommerce to modify the fields to a textarea, select, etc.
Save the New Checkout Fields Upon Successful Order
Now that our fields are displaying properly we need to save them to the order meta when the order goes through. We will again use a WooCommerce hook to do this called woocommerce_checkout_update_order_meta.
Add the following code below the function provided above and update with your necessary modifications:
/** [3] Update the order meta with field value **/
add_action( 'woocommerce_checkout_update_order_meta', 'icw_custom_checkout_field_update_order_meta' );
function icw_custom_checkout_field_update_order_meta( $order_id ) {
//check if $_POST has our custom fields
if ( $_POST['ticket-pickup'] ) {
//It does: update post meta for this order
update_post_meta( $order_id, 'A) Ticket(s) Pickup', esc_attr( $_POST['ticket-pickup'] ) );
}
if ( $_POST['if-checked-address-tickets'] ) {
update_post_meta( $order_id, 'A) If tickets address', esc_attr( $_POST['if-checked-address-tickets'] ) );
}
if ( $_POST['if-checked'] ) {
//It does: update post meta for this order
update_post_meta( $order_id, 'B) Is attendees under 21?', 'Yes' );
}
if ( $_POST['if-checked-under-21'] ) {
update_post_meta( $order_id, 'B) How many under 21 will be attending?', esc_attr( $_POST['if-checked-under-21'] ) );
}
}
Now test an order out and you should see our custom field data in the order’s post meta:
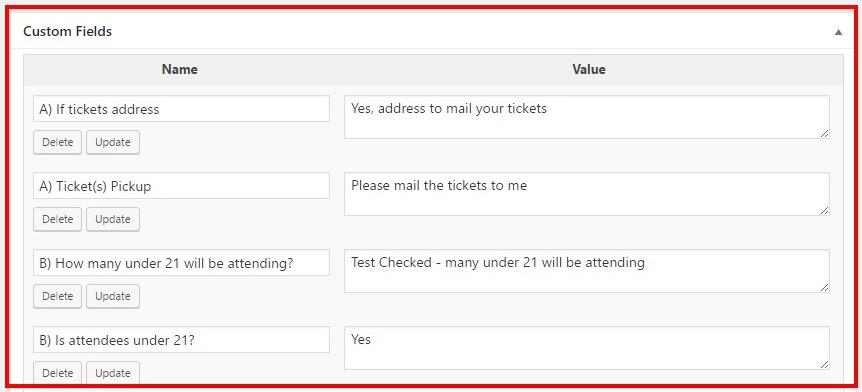
Now that the data is attached to the post you can do whatever you like with it.
Add the Custom Field Data to Order Emails
Most likely the shop owner is notified of orders via email. What we want to do is send our custom field data along with that email so they can fulfill the order accordingly.
We are going to be using a filter called woocommerce_email_order_meta_keys to send our data along with the email. Again, we will first check the the order does contain the product because we don’t want to clutter up the email with blank custom fields.
/** [4] Add the field to order emails **/
add_filter( 'woocommerce_email_order_meta_keys', 'icw_checkout_field_order_meta_keys' );
function icw_checkout_field_order_meta_keys( $keys ) {
//Check if Book in Cart
$ticket_pickup_in_cart = icw_is_conditional_product_in_cart( 1235 ); // enter product id @icw
//Only if book in cart
if ( $ticket_pickup_in_cart === true ) {
$keys[] = 'A) Ticket(s) Pickup';
$keys[] = 'A) If tickets address';
$keys[] = 'B) Is attendees under 21?';
$keys[] = 'B) How many under 21 will be attending?';
}
return $keys;
}
Now when an order goes that contains our specific product, in this case a book, our fields will display:
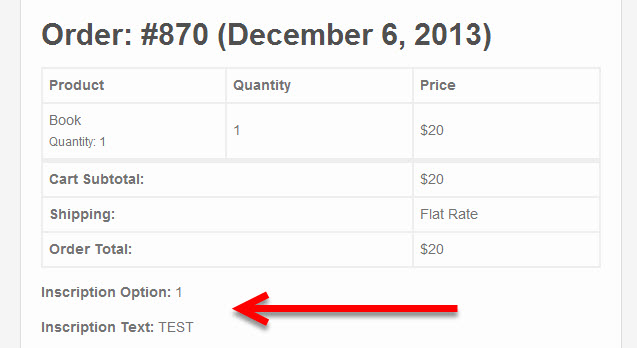
Conditional Checkout Fields: Oh the Potential
With conditional checkout fields in WooCommerce there is a lot of potential use cases. For instance, you can ask a user to sign up to a specific mailing list based on their product choice, ask for additional shipping requirements or special handling questions, or request additional information from the user. The system is setup to be very flexible, you just have to know how to use it.
Author
Jayesh Patel
Jayesh Patel is a Professional Web Developer & Designer and the Founder of InCreativeWeb.
As a highly Creative Web/Graphic/UI Designer - Front End / PHP / WordPress / Shopify Developer, with 14+ years of experience, he also provide complete solution from SEO to Digital Marketing. The passion he has for his work, his dedication, and ability to make quick, decisive decisions set him apart from the rest.
His first priority is to create a website with Complete SEO + Speed Up + WordPress Security Code of standards.